In this blog post, we will look into the basic difference in Blazor Server and WebAssembly Application and will point out the difference in their default project structure and code differences, advantages, and disadvantages of using different application types, etc. If you are completely new to Blazor, I would highly recommend you go through the introductory post.
Blazor is a new framework provided by Microsoft to build interactive client-side web applications using C# programming language and Razor syntax on top of the .NET Core framework. The beauty of this framework is that the developer need not know any JavaScript and can leverage the existing .NET, .NET Core frameworks, and their associated NuGet packages to power the Blazor application. The developer can build a Blazor application that can run on the server or directly inside the client browsers.
The difference in Blazor Server and WebAssembly Application
Below given is the list of 6 major difference in Blazor Server and WebAssembly Application, let us see each one in brief.
Hosting Models
The Blazor Hosting Model defines the type of Blazor Application. If the Blazor application is hosted under the ASP.NET Core Application on the server, then it is termed as Blazor Server Application. In this type of application all the UI updates, user interactions are executed on the Server using SignalR connection. IF the connection is lost then the application stops working.
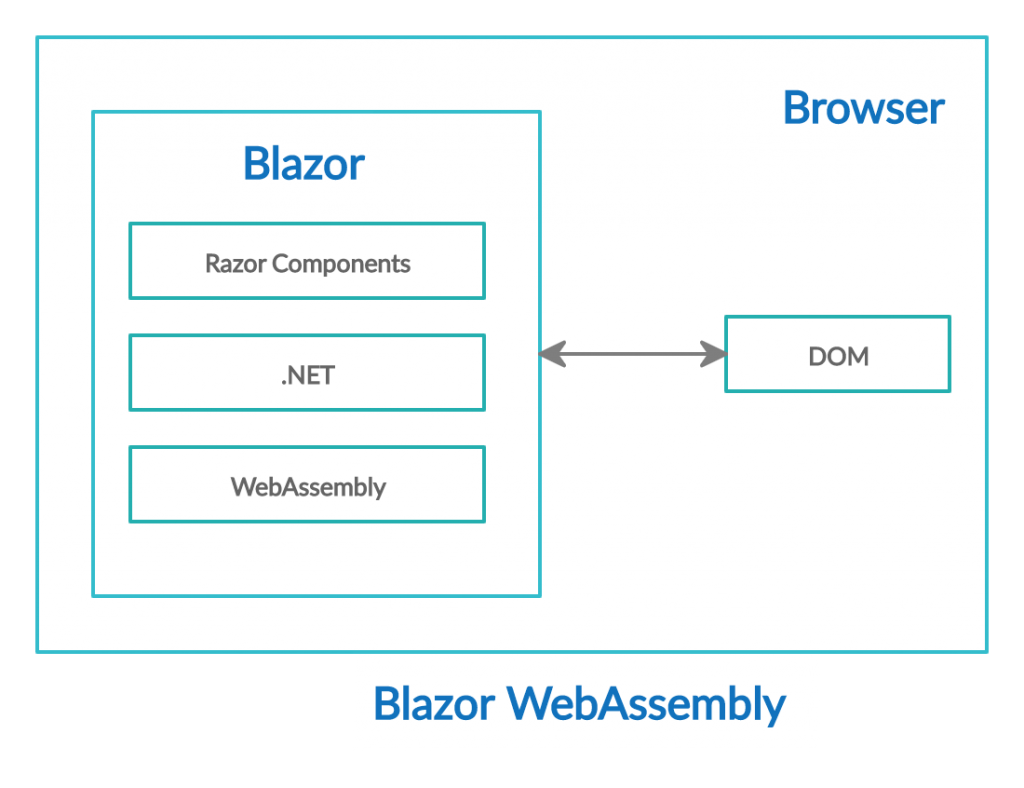
If the Blazor Application is downloaded and hosted in the client’s browser, then it is termed as Blazor WebAssembly Application. The complete application along with dependencies is downloaded and it executes without the need of any server under the defined browser’s limitations.
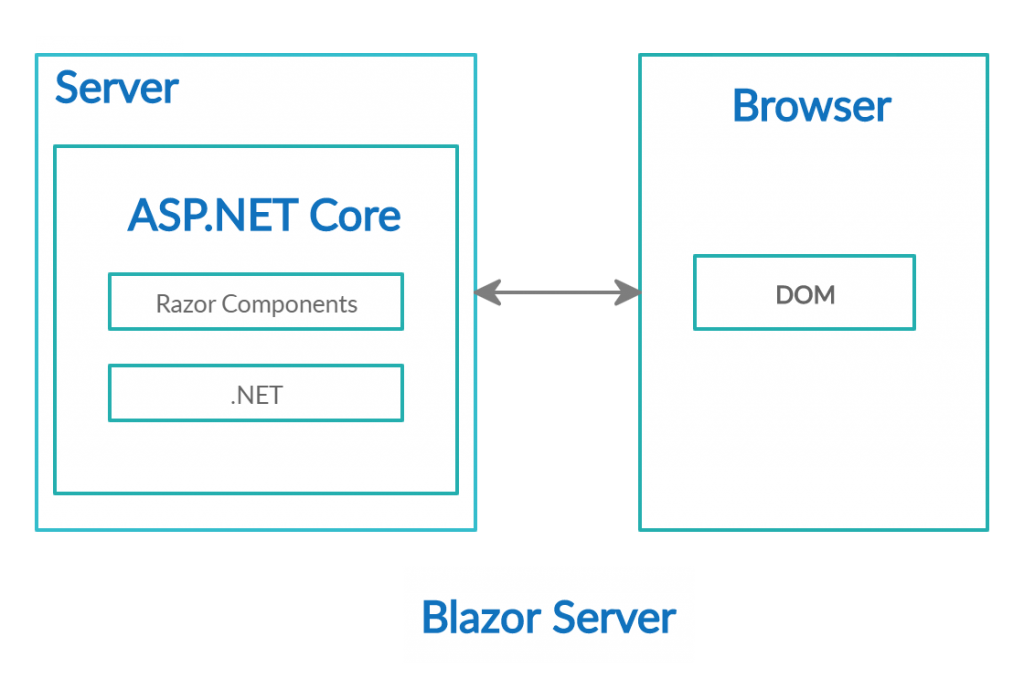
Project Structure Differences
The below snapshot displays the project structure of Blazor Server and Blazor WebAssembly applications. Both projects have been created in Visual Studio 2019 using .NET Core v3.1 as the underlying framework. The differences in the files and layout are detailed below:
File present only in Blazor Server App
- WeatherForecast.cs
- WeatherForecastService.cs
- appsettings.Development.json
- appsettings.json
- Startup.cs
- _Host.cshtml
File present only in WebAssembly App
- index.html
- weather.json
Blazor Server site.css equivalent file app.css is available in WebAssembly.
The JavaScript Reference
- _framework/blazor.webassembly.js: This JavaScript is referenced in the index.html file and is responsible for downloading all the statics files, .NET Core Assemblies, and the WebAssembly version of the .NET Core runtime required by the application. The JavaScript also initializes the runtime to execute the application.
- _framework/blazor.server.js: This JavaScript is referenced in the _Host.cshtml file and is responsible for establishing the client’s Web Socket connection with the server.
Application Initialization
- Startup.cs: The Startup class is responsible for initializing the services required to execute the Blazor Server application. It is also responsible for loading the application configurations as well as initializing the SignalR Hub for the Web Socket connection required by the client.
namespace BlazorServerApp { public class Startup { public Startup(IConfiguration configuration) { Configuration = configuration; } public IConfiguration Configuration { get; } // This method gets called by the runtime. Use this method to add services to the container. // For more information on how to configure your application, visit https://go.microsoft.com/fwlink/?LinkID=398940 public void ConfigureServices(IServiceCollection services) { services.AddRazorPages(); services.AddServerSideBlazor(); services.AddSingleton<WeatherForecastService>(); } // This method gets called by the runtime. Use this method to configure the HTTP request pipeline. public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } else { app.UseExceptionHandler("/Error"); // The default HSTS value is 30 days. You may want to change this for production scenarios, see https://aka.ms/aspnetcore-hsts. app.UseHsts(); } app.UseHttpsRedirection(); app.UseStaticFiles(); app.UseRouting(); app.UseEndpoints(endpoints => { endpoints.MapBlazorHub(); endpoints.MapFallbackToPage("/_Host"); }); } } }
- Program.cs: The Blazor WebAssembly application does not contain Startup.cs, the initialization of the WebAssemblyHostBuilder, loading of the root App component, and executing the application is handled in the Program.cs file.
namespace BlazorWebAssemblyApp { public class Program { public static async Task Main(string[] args) { var builder = WebAssemblyHostBuilder.CreateDefault(args); builder.RootComponents.Add<App>("app"); builder.Services.AddTransient(sp => new HttpClient { BaseAddress = new Uri(builder.HostEnvironment.BaseAddress) }); await builder.Build().RunAsync(); } } }
Advantages
Advantages of Blazor Server Application
- The application executes within ASP.NET Core runtime on the server, hence all the .NET Core API is available for use along with its tooling capabilities.
- The Server only serves HTML and JavaScript to the client, not the .NET codebase.
- Significantly smaller download size and the application load much faster compared to WebAssembly.
- Thin client support, the application can be used with all types of browsers with or without WebAssembly support.
Advantages of Blazor WebAssembly Application
- The application can leverage the power of CPU and can give an almost native app like performance.
- The application does not require the server to perform any of its operations. Once downloaded, the application is fully functional.
- As no ASP.NET Core Web Server is required to execute the application, it can be distributed using CDN making server-less deployment possible.
Disadvantages
Disadvantages of Blazor Server Application
- Every user interaction requires a trip to the server.
- The application will stop working if the connection is lost with the server.
- Handling multiple users is challenging as the server needs to manage multiple client connections and client states.
- ASP.NET Core server is required to serve the application making server-less deployment not possible.
Disadvantages of Blazor WebAssembly Application
- The application takes a long time to load as the download size is larger.
- The application is restricted as per browser capabilities.
- A browser supporting WebAssembly can only execute the application.
- Debugging capabilities are currently limited.
This concludes the post, I hope you find the difference in Blazor Server and WebAssembly Application helpful. Thanks for visiting, Cheers!!!.
The other tools and packages required for building Blazor Application can be downloaded from the given link
- Visual Studio 2019 (v16.4) and above [download here]
- .NET Core SDK 3.1 and above [download here]
[Further Readings: Exploring Blazor WebAssembly App Project Structure | Top 10 Productivity Tips and Tricks in Visual Studio 2019 | CRUD Operations in WPF using EntityFrameworkCore and SQLite | How to implement Dependency Injection in WPF | How to use External Tool in Visual Studio 2019 | Top Features of Visual Studio 2019 Community Edition | Basic CRUD operations in Blazor using SQLite as the database | How to consume REST API in Blazor Application | Blazor Lifecycle Methods | A Simple way to Call Javascript in Blazor Application | Creational Design Patterns | Builder Design Pattern in C# | Prototype Design Pattern in C# | Top 5 Blazor Component Libraries | Abstract Factory Design Pattern in C# | Factory Method Pattern in C# | Singleton Design Pattern in C# | Introduction to Design Patterns ]