The Blazor framework provides synchronous and asynchronous versions of application lifecycle methods and can be used to perform additional initialization and checks on the component. In this blog, we are going to look into these Blazor lifecycle methods and understand how and when they are called.
Blazor is a new framework provided by Microsoft to build interactive client-side web applications using C# programming language and Razor syntax on top of the .NET Core framework. The beauty of this framework is that the developer need not know any JavaScript and can leverage the existing .NET, .NET Core frameworks and its associated NuGet packages to power the Blazor application. The developer can build a Blazor application that can run on the server or directly inside the client browsers.
Blazor Application Lifecycle Methods
The Blazor application lifecycle methods provide a way to execute additional functionality or operations on a Razor component while it is being initialized and being rendered. The following are the lifecycle methods that are executed during the initializing and rendering of the component.
- SetParametersAsync()
- OnInitialized()
- OnInitializedAsync()
- OnParametersSet()
- OnParametersSetAsync()
- OnAfterRender(bool firstRender)
- OnAfterRenderAsync(bool firstRender)
- ShouldRender()
As we can see in the above method list every method has its asynchronous version. This asynchronous version is executed only after the synchronous version has completed its execution.
Blazor Lifecycle Methods – Flow and Explanation
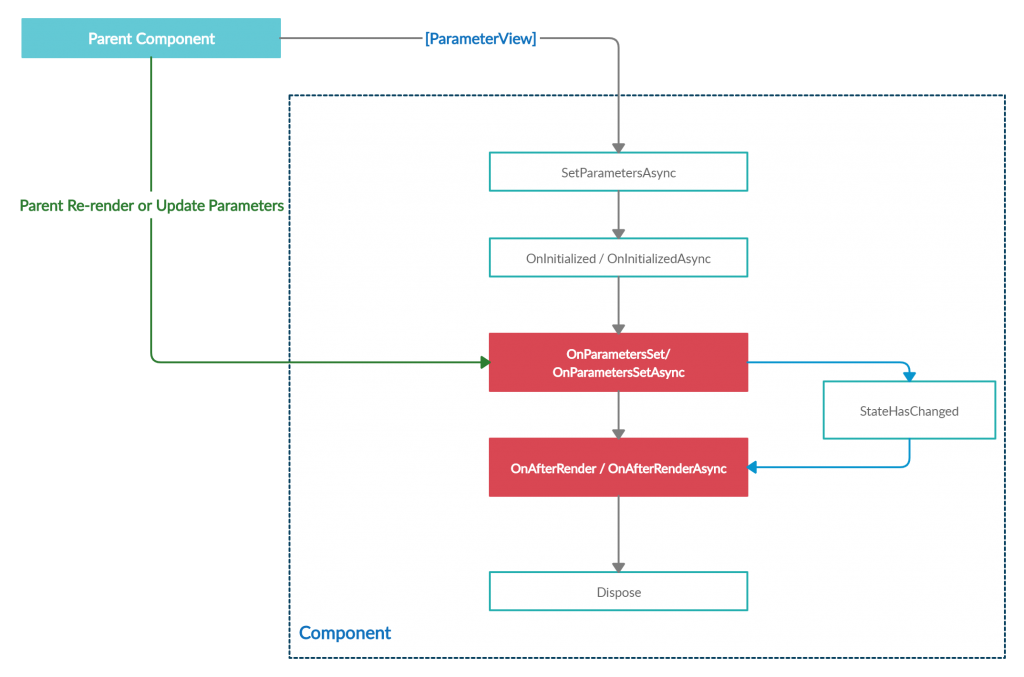
Let us discuss each of the method in details below:
SetParametersAsync
SetParametersAsync is the first method that is executed when the component is created. This method sets the parameters that are provided by the parent of the component in the rendering tree. The method provides the parameter called ParameterView which contains all the values for every property with the [Parameter] or [CascadingParamter] attribute.
OnInitialized and OnInitializedAsync
The OnInitialized and OnInitializedAsync methods as its name suggests is invoked when the component is being initialized. These methods are invoked when the parent component has passed the initial parameter to the initializing component.
These methods are the ideal place to call any data services required by the component. And if the requirement is to refresh the UI after the data is loaded, calling the asynchronous version is the place to do so.
OnParametersSet and OnParametersSetAsync
The OnParametersSet and OnParametersSetAsync methods are called when the component has received parameters from its parent component and these values are assigned to its properties. These methods are invoked whenever the parent component re-renders or new or parameters are updated by its parent component which in turn executes the OnAfterRender and OnAfterRenderAsync methods.
OnAfterRender and OnAfterRenderAsync
The OnAfterRender and OnAfterRenderAsync methods are called after the component has finished rendering. At this point, all the elements and the component references are populated. The methods can be used to perform additional initialization of the component, such as event attaching an event listener or initializing the JavaScript libraries which require the DOM elements to be present in order to work.
These methods have “firstRender” parameter that is set to true only for the first time when the component instance is rendered and can be used to make sure that the initialization of the component is done only once.
ShouldRender
The ShouldRender method is invoked right before the component is re-rendered and can be used to stop the current and future rendering of the component. If the method returns true the UI is refreshed else the refreshing is suppressed.
StateHasChanged
This is a special method that does not belong to the Blazor lifecycle methods group but is used to notify that the state of the component has changed and asking the framework to refresh the UI.
Code to display Blazor Lifecycle Methods
The sample code to display the invocation of the method.
@page "/" <div class="container"> <h3>Blazor - Application Lifecycle Method</h3> </div> <br /><br /> @foreach (var item in LifeCycleEvents) { @item <hr /> } @code { public List<string> LifeCycleEvents = new List<string>(); protected override void OnInitialized() { LifeCycleEvents.Add("OnInitialized ..."); } protected override async Task OnInitializedAsync() { LifeCycleEvents.Add("OnInitializedAsync ..."); await Task.Delay(500); } protected override void OnParametersSet() { LifeCycleEvents.Add("OnParametersSet ..."); } protected override async Task OnParametersSetAsync() { LifeCycleEvents.Add("OnParametersSetAsync ..."); await Task.Delay(500); } protected override void OnAfterRender(bool firstRender) { LifeCycleEvents.Add("OnAfterRender [FirstRender]..."); } protected override async Task OnAfterRenderAsync(bool firstRender) { LifeCycleEvents.Add("OnAfterRenderAsync [FirstRender] ..."); await Task.Delay(500); } protected override bool ShouldRender() { LifeCycleEvents.Add("ShouldRender ..."); return true; } public override async Task SetParametersAsync(ParameterView parameters) { LifeCycleEvents.Add("SetParametersAsync [Parameter View] ..."); await base.SetParametersAsync(parameters); await Task.Delay(500); } }
Output of the code
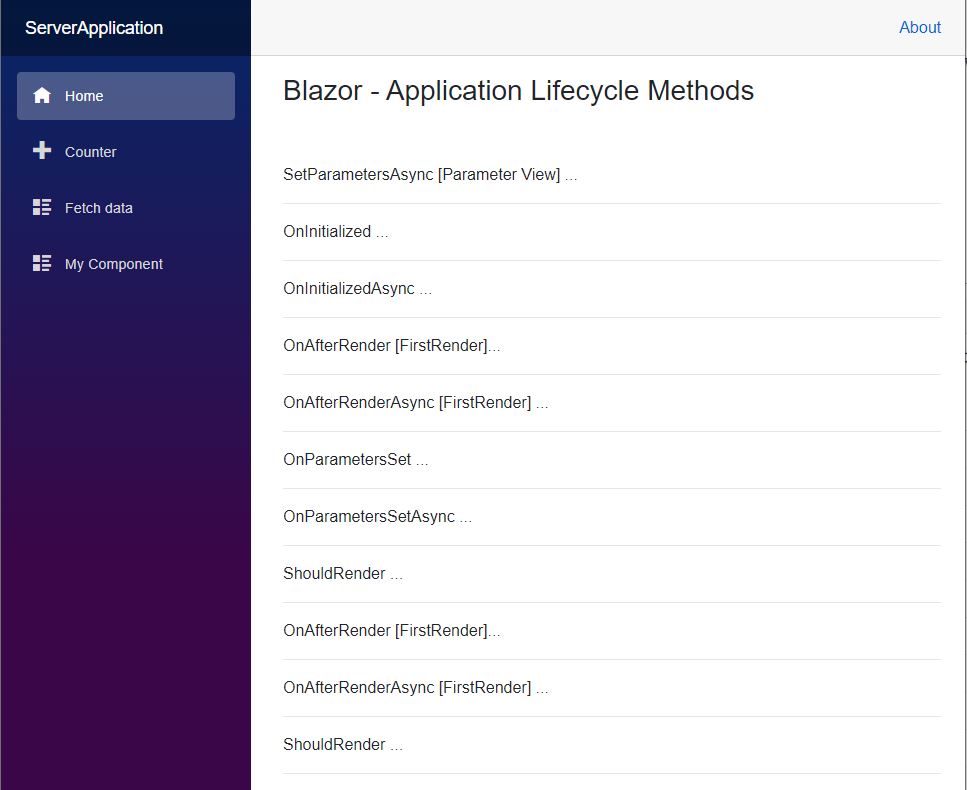
This concludes the post about the Blazor Lifecycle Methods and I hope you found this post helpful. Thanks for visiting, Cheers!!!
[Further Readings: A Simple way to Call Javascript in Blazor Application | Creational Design Patterns | Builder Design Pattern in C# | Prototype Design Pattern in C# | Top 5 Blazor Component Libraries | Abstract Factory Design Pattern in C# | Factory Method Pattern in C# | Singleton Design Pattern in C# | Introduction to Design Patterns | Microsoft C# Version History | Microsoft .NET Core Versions History | Microsoft .NET Framework Version History | Introduction to WPF in .NET Core | Useful Visual Studio 2019 extensions for database projects | Machine Learning Model Generation ]